LeetCode 솔루션 분류
[8/12] 235. Lowest Common Ancestor of a Binary Search Tree
본문
Easy
6840214Add to ListShareGiven a binary search tree (BST), find the lowest common ancestor (LCA) node of two given nodes in the BST.
According to the definition of LCA on Wikipedia: “The lowest common ancestor is defined between two nodes p
and q
as the lowest node in T
that has both p
and q
as descendants (where we allow a node to be a descendant of itself).”
Example 1:
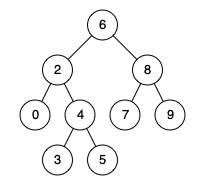
Input: root = [6,2,8,0,4,7,9,null,null,3,5], p = 2, q = 8 Output: 6 Explanation: The LCA of nodes 2 and 8 is 6.
Example 2:
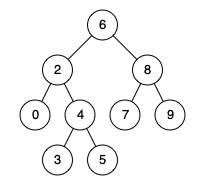
Input: root = [6,2,8,0,4,7,9,null,null,3,5], p = 2, q = 4 Output: 2 Explanation: The LCA of nodes 2 and 4 is 2, since a node can be a descendant of itself according to the LCA definition.
Example 3:
Input: root = [2,1], p = 2, q = 1 Output: 2
Constraints:
- The number of nodes in the tree is in the range
[2, 105]
. -109 <= Node.val <= 109
- All
Node.val
are unique. p != q
p
andq
will exist in the BST.
Accepted
879,547
Submissions
1,503,941
관련자료
-
링크
댓글 2
학부유학생님의 댓글
- 익명
- 작성일
Runtime: 146 ms, faster than 25.90% of Python3 online submissions for Lowest Common Ancestor of a Binary Search Tree.
Memory Usage: 18.8 MB, less than 22.99% of Python3 online submissions for Lowest Common Ancestor of a Binary Search Tree.
Memory Usage: 18.8 MB, less than 22.99% of Python3 online submissions for Lowest Common Ancestor of a Binary Search Tree.
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def lowestCommonAncestor(self, root: 'TreeNode', p: 'TreeNode', q: 'TreeNode') -> 'TreeNode':
if not root: return None
if root == p or root == q: return root
left = self.lowestCommonAncestor(root.left, p, q)
right = self.lowestCommonAncestor(root.right, p, q)
if left != None and right != None: return root
if left != None: return left
if right != None: return right
재민재민님의 댓글
- 익명
- 작성일
Runtime: 34 ms, faster than 86.93% of C++ online submissions for Lowest Common Ancestor of a Binary Search Tree.
Memory Usage: 23.2 MB, less than 91.99% of C++ online submissions for Lowest Common Ancestor of a Binary Search Tree.
Memory Usage: 23.2 MB, less than 91.99% of C++ online submissions for Lowest Common Ancestor of a Binary Search Tree.
class Solution {
public:
TreeNode* lowestCommonAncestor(TreeNode* root, TreeNode* p, TreeNode* q) {
int s_val = min(p->val, q->val);
int l_val = max(p->val, q->val);
if(s_val == root->val || root->val == l_val)
return root;
else if(s_val < root->val && root->val < l_val)
return root;
else if(s_val < root->val && l_val < root->val )
return lowestCommonAncestor(root->left, p, q);
else if(s_val > root->val && l_val > root->val )
return lowestCommonAncestor(root->right, p, q);
return NULL;
}
};