LeetCode 솔루션 분류
538. Convert BST to Greater Tree
본문
538. Convert BST to Greater Tree
Medium
3226149Add to ListShareGiven the root
of a Binary Search Tree (BST), convert it to a Greater Tree such that every key of the original BST is changed to the original key plus the sum of all keys greater than the original key in BST.
As a reminder, a binary search tree is a tree that satisfies these constraints:
- The left subtree of a node contains only nodes with keys less than the node's key.
- The right subtree of a node contains only nodes with keys greater than the node's key.
- Both the left and right subtrees must also be binary search trees.
Example 1:
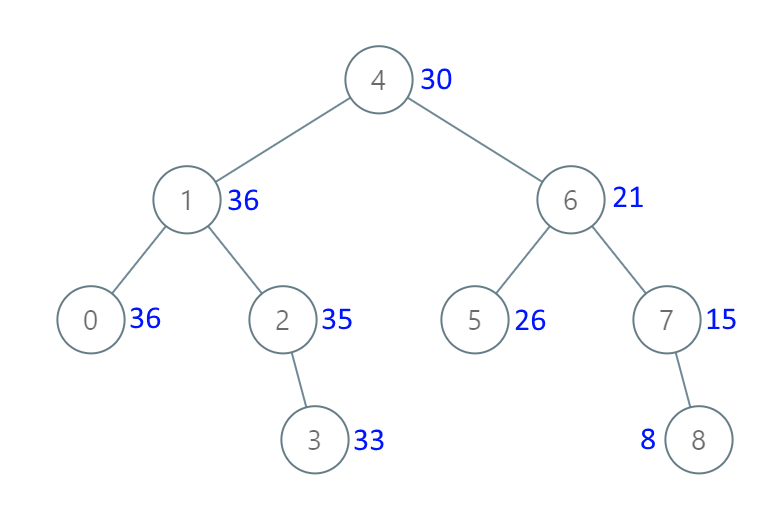
Input: root = [4,1,6,0,2,5,7,null,null,null,3,null,null,null,8] Output: [30,36,21,36,35,26,15,null,null,null,33,null,null,null,8]
Example 2:
Input: root = [0,null,1] Output: [1,null,1]
Constraints:
- The number of nodes in the tree is in the range
[0, 104]
. -104 <= Node.val <= 104
- All the values in the tree are unique.
root
is guaranteed to be a valid binary search tree.
Note: This question is the same as 1038: https://leetcode.com/problems/binary-search-tree-to-greater-sum-tree/
관련자료
-
링크
댓글 2
mingki님의 댓글
- 익명
- 작성일
C++
Runtime: 36 ms, faster than 90.34% of C++ online submissions for Convert BST to Greater Tree.
Memory Usage: 33.5 MB, less than 62.62% of C++ online submissions for Convert BST to Greater Tree.
Runtime: 36 ms, faster than 90.34% of C++ online submissions for Convert BST to Greater Tree.
Memory Usage: 33.5 MB, less than 62.62% of C++ online submissions for Convert BST to Greater Tree.
class Solution {
private:
int sum = 0;
public:
TreeNode* convertBST(TreeNode* root) {
if (root) {
convertBST(root->right);
root->val = (sum += root->val);
convertBST(root->left);
}
return root;
}
};
bobkim님의 댓글
- 익명
- 작성일
Runtime: 51 ms, faster than 22.70% of C++ online submissions for Convert BST to Greater Tree.
Memory Usage: 33.5 MB, less than 19.98% of C++ online submissions for Convert BST to Greater Tree.
Memory Usage: 33.5 MB, less than 19.98% of C++ online submissions for Convert BST to Greater Tree.
class Solution {
public:
void sumBST(TreeNode* &p, int &sum){
if(p->right != nullptr)
sumBST(p->right,sum);
p->val = sum + p->val;
sum=p->val;
if(p->left != nullptr)
sumBST(p->left,sum);
};
TreeNode* convertBST(TreeNode* root) {
int sum=0;
if(root!=nullptr){
sumBST(root,sum);
};
return root;
}
};